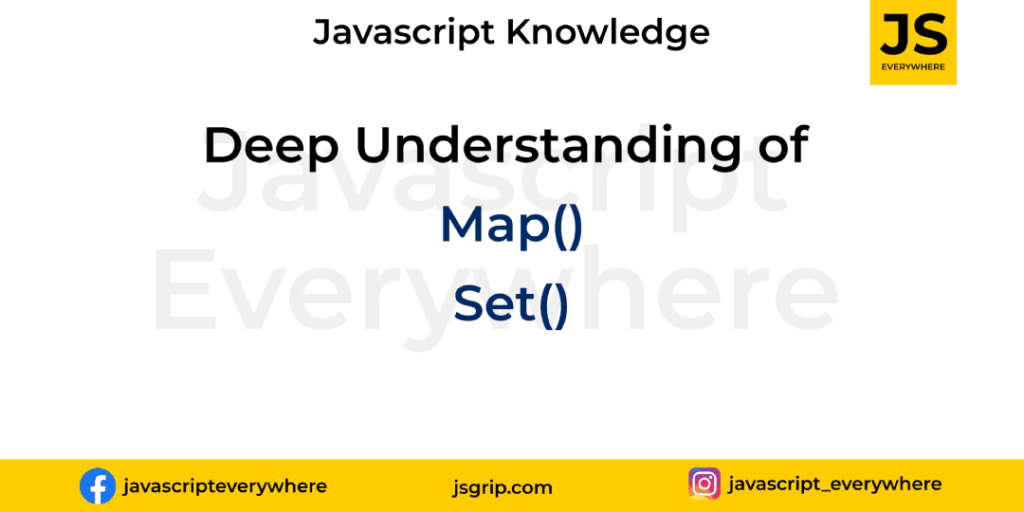
The Map object store key-value pairs and remembers the original insertion order of the keys
The set object store unique value of any types, It’s maybe primitive or object types
Map
Map does not content any default keys.
let testMap = new Map();
console.log(testMap);
In The Map key can be any value like array, string, symbol, function, object or any other. And Object contain only string or symbol
let testMap = new Map();
const functionTest = () => {
console.log('Hello')
}
const arrayTest = [1, 2, 3, 4];
const testObj = {name: 'Javascript Everywhere'};
testMap.set(functionTest, "test Function Data here");
testMap.set(arrayTest, "Array as key with value");
testMap.set(testObj, "Object as key for map");
console.log(testMap.get(functionTest));
console.log(testMap.get(arrayTest));
console.log(testMap.get(testObj));
The key of map are ordered. It’s insertion order.
Many function provide for map, Size property is return number of item in map. And number of items in object you should determine manually
const mapData = new Map();
mapData.set('a', "First Value");
mapData.set('b', "Second Value");
mapData.set('c', "third Value");
mapData.set('d', "fourth Value");
mapData.set('e', "fifth Value");
console.log(mapData.size);
A Map is an iterable. For iteration you can click and read this.
// Take above example as object Data
mapData.forEach(function(key, value){
console.log(key, value);
});
You can not add new key in map as you add object key.
const checkData = new Map();
checkData['asObjectKey'] = 'As Object Data';
checkData.set('data', 'Test Javascript everywhere Data');
console.log(checkData.has('data')) // Return true
console.log(checkData.has('asObjectKey')) // Return false
How to remove all key value pairs from map?
Answer: using clear() function you can remove all item from map
How to remove specific key-value from map?
Answer: Using delete(key) function you can remove item from map
How to get value from map?
Answer: Using get(key) function you can retrieve the value from the map. A key is not present then return undefined
How to check key is existing or not?
Answer: using has(key) function you check object is present or not. It’s return boolean.
How will we get all keys from map?
Answer: keys() function return list of key from the map
How to get all values from map?
Answer: Using values() function you an get all values in array
Can we merge two maps?
Answer: yes we can do it, let’s take one example.
Merge two maps. More than two key give in merge then accept the last one is final. Spread operator essentially converts a Map to an Array
let mapFirst = new Map([
['a', 'js'],
['b', 'javascript'],
['c', 'jsgrip.com'],
])
let mapSecond = new Map([
['d', 'Welcome to best website'],
['e', 'Hello Developer']
])
let merged = new Map([...mapFirst, ...mapSecond]);
console.log(merged.get('a'))
console.log(merged.get('e'))
You can use NaN as key as well
const checkData = new Map();
checkData.set(NaN, 'Null Value');
console.log(checkData.get(NaN)); // Display "Null Value"
Set
A Set is collection of values. Set has unique value.
let testSetData = new Set();
testSetData.add('a');
testSetData.add(function(){});
testSetData.add(2);
testSetData.add("Javascript everywhere");
Remove duplicate elements from the array
// Use to remove duplicate elements from the array
const numbers = [2,3,1,1,2,3,3,4,4,5,5,6,6,7,5,'1',3,'1','a', 'b']
console.log([...new Set(numbers)])
Passing string inside set is consider as array set and remove duplicate with case sensitive
let jsText = 'Javascript Everywhere'
//case sensitive & duplicate omit
let mySet = new Set(jsText)
console.log(mySet.size) // 15
console.log(...mySet) // J a v s c r i p t E e y w h
size property use for getting a count of values in the set
add(value) function is use for add new value in the set
clear() method use for clear all value from the set
delete(value) function is used for delete element from a set
has(value) function check value exist or not
If you have any question regarding map() and set() you can contact as using contact page