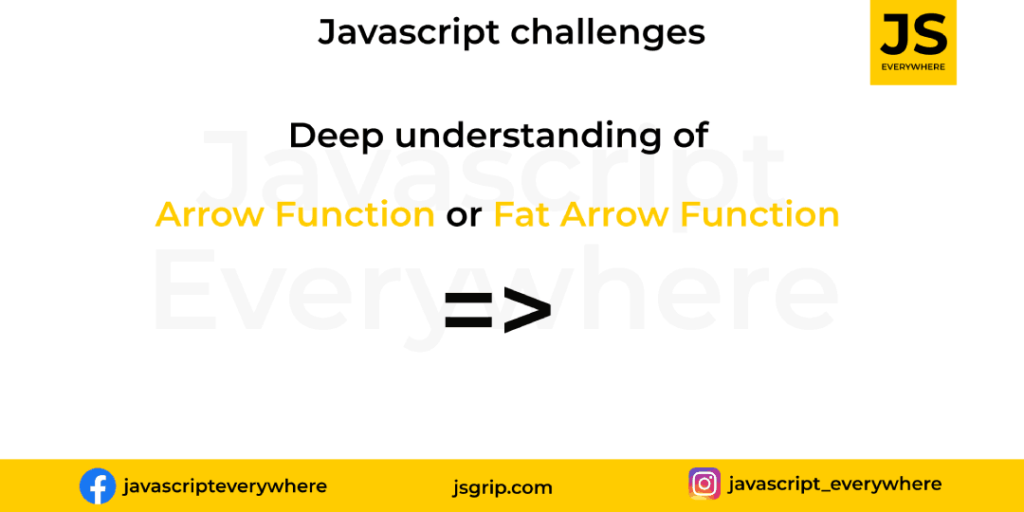
The best feature ever release by javascript is the Arrow function. An arrow function is also known as a fat arrow function. Basically the use of arrow function is a concise way to write the function in javascript.
In many languages like c++, python, ruby or any other provide us to arrow use. Some developers say mini function as well or lambda or scope many words were spoken by the developer.
We love JavaScript. Because JavaScript also provide arrow function to help developer
Arrow function are avoid function and return keyword.
There are variety of syntax for fat function. We cover most use full and common syntax here.
Basic Syntax
var testFunction = () => {
// Write your logic here
};
testFunction is name of function here no need to write function keyword.
Let’s take one small example and understand how we use in or real life problem.
//ES5
function jsOldFunction(val1,val2) {
return val1 + val2;
}
console.log(jsOldFunction(5, 5));
// ES6
var jsFunction = (value1, value2) => { return value1 + value2 };
console.log(jsFunction(10, 20));
Is it clear? Many Way to use arrow function so we will cover one by one with example as well
No parameter
const myPageName = () => {console.log('Javascript everywhere')}
myPageName();
It’s very easy, just pass empty parenthesis with => Even {} this is also not required
With a single parameter
When you are going to create arrow function no need to write parenthesis as well it very funny and useful
//const printWebsiteName = name => {console.log(name)};
const printWebsiteName = name => console.log(name);
printWebsiteName('jsgrip.com');
Function with return block
Sometimes we need return build blog as JSON or any other so we can use below syntax for it.
let jsonPre = (name, like) => ({name: name, like: like});
console.log(jsonPre("javascript everywhere", 965865));
//{
// name:"javascript everywhere",
// like:965865
//}
Without parenthesis it’s give an error.
Let compare both syntax old and new with example
let facebookPages = [
{name: "javascript everywhere", followers: 98465681},
{name: "Javascript", followers: 5252},
{name: "Unknown page", followers: 981},
];
const data = facebookPages.map(function(fb){
return fb.followers
});
console.log(data);
let fbData = facebookPages.map(element => element.followers);
console.log(fbData);
Don’t be confuse both function has same output just syntax is different.
Js Developer ask me a question can we use arrow function with promises and callback?
And my answer is Yes we can use it.
// ES5
oneAsync().then(function() {
// code here
}).then(function() {
// resolve or faild
}).done(function() {
// Last line of output
});
// ES6
oneAsync().then(() => code here).then(() => resolve or faild).done(() => Last);
For understanding promise or Async/await function.
Keep some point in your mind for arrow function
- Don’t use curly brackets if only one statement.
- You can omit return as well if a single statement in the function
- No need to use parenthesis is only a single argument in the function.
If any confusion related to Arrow function you can ask us to any time.
Thanks for good explanation and keep me in list for next posts.
Great
Very nice
Great Post on Arrow function.