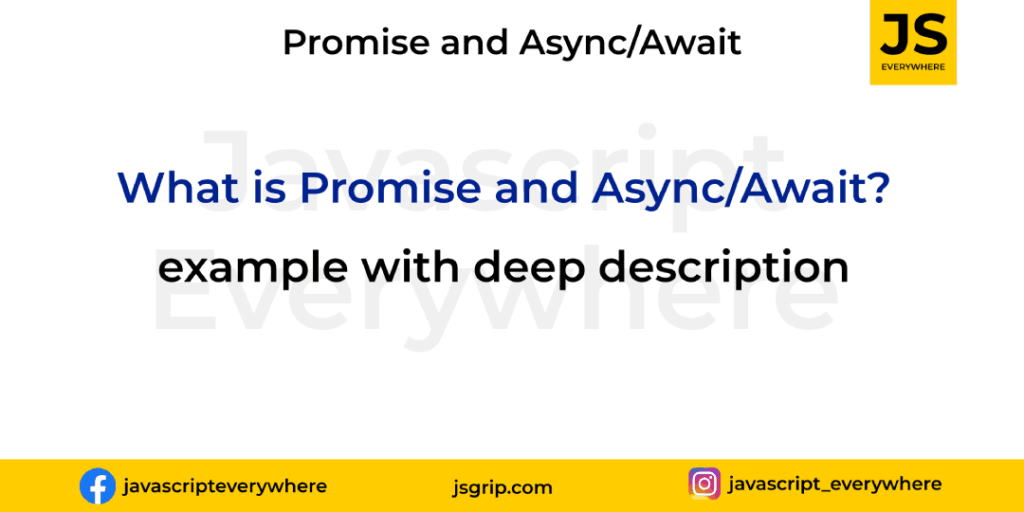
Base on developer requirement we are going to cover promises and async/await with this article. Some developers are still confused about how to use promises and async javascript. JavaScript Promises are very similar to real-life promises made by us. After that promises, we make a plan and assure that we are successfully have done or broke.
JS promises are completion or failure.
Mainly two-part of promises in javascript:
1) Create a promise
2) Handing a promise
How to create promise?
new Promise(function(resolve, reject){
// implement logic here
})
Promise function accept two parameter resolve and reject.
Which is best place to use promise?
- Promise use for database transition like bank transactions or any other.
- Open the file and write or modify on it like I/O operation
- During APIs call it to wait until API not responding.
After all these operation promise are resolve or reject base on success response or error
let promise = new Promise(function(resolve, reject) {
if(condition) {
resolve("done");
} else {
reject("error");
}
});
As we see javascript execute immediately but the promise is waiting for the success or failure
and then return the response.
Based on above discussion promise has three state, pending, success and reject.
Now let’s take one example
let promise = new Promise(function(resolve, reject) {
setTimeout(function(){
console.log("Javascript everywhere");
}, 5000);
})
console.log(promise)
// OR
promise.then(res => { console.log('success)}).catch(error => {console.log('error')})
console.log() is execute after 5 seconds
There is chain of promise also possible but if single promise failed in between all other are also failed.
let promise1 = new Promise(function(res, reject){
console.log('promise 1 is running');
res('done');
})
promise1.then(res1 => {
console.log('Start promise 2 run success');
}).then(res2 => {
console.log('start promise 3 Javascript everywhere');
}).then(res3 => {
console.log("start promise 4 JSGrip");
})
JavaScript is a synchronous language.There is many way JavaScript behave like an asynchronous language one of them is async-await
Async/Await
Async/Await are extensions of javascript promises.
function promiseTest() {
return new Promise(resolve => {
setTimeout(() => {
resolve('Javascript everywhere for developer');
}, 2000);
});
}
async function testPromise() {
const p1 = await promiseTest();
}
testPromise(); // Display after 2 second
Async is a function that enable us to write promise code with out blocking the execution of other thread.Async function always return a value. If promise is not return then javascript promise block it self.
function promise1() {
return new Promise(resolve => {
setTimeout(() => {
resolve("First promise resolve");
}, 1000);
});
}
function promise2() {
return new Promise(resolve => {
setTimeout(() => {
resolve('Second promise resolve');
}, 500);
});
}
function promise3() {
return new Promise(resolve => {
setTimeout(() => {
resolve('Third promise resolve');
}, 2000);
});
}
async function resolvePromise() {
const p1 = await promise1();
const p2 = await promise2();
const p3 = await promise3();
console.log(p1);
console.log(p2);
console.log(p3);
}
resolvePromise(); // Run after 2.5 second
// OR
async function resolvePromise() {
const [p1, p2, p3] = await Promise.all([promise1(), promise2(), promise3()]);
console.log(p1);
console.log(p2);
console.log(p3);
}
resolvePromise(); // Run after 2.5 second
The above example is waiting for all promise and until all are not done output not display. Whatever response comes from it may succeed or fail as well.
Now you job is test promise, async/await with variable access between promise or not.
Most of developer use asynchronous JavaScript for calling API and open file and operation related to database.
async function fetchCounty(endpoint) {
const res = await fetch(endpoint);
let data = await res.json();
data = data.map(country => country.name);
console.log(data);
}
fetchCounty('https://yousiteurlforapi/country');
And wait for our new challenge come including promise and async/await.
Note: Internet explorer is not support Async functions
This explanation is full clear. But i don’t understand how to use subscription.
Can you explain in detail, my friend?