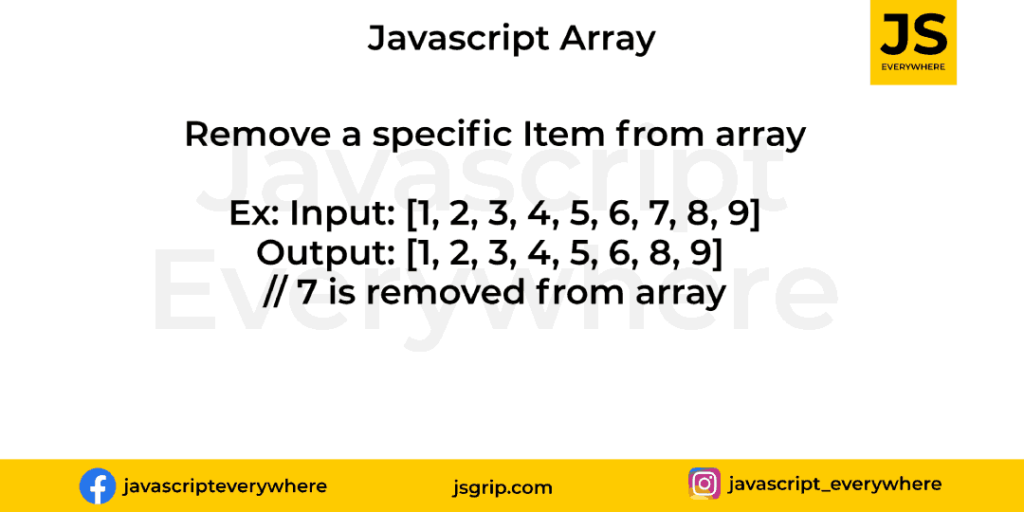
A common question asked by the developer “How to remove a specific item from an array?”. Many developers ask questions related to an array. Some developers are beginners in our network, So we will try to clear the JavaScript concept using the article or direct communication.
Let’s deep dive into Javascript array.
The array is a single element that store or hold multiple-element with different datatype as well. Here different datatype means a single array store string, integer, float, object, and JSON. for easy understanding let take a small example.
function testData() {
return 'function call inside array';
}
let testArray = [1, 2, 3, 'javascript everywhere', "jsgrip", {a: 1, obj: 'developer'}, 1.2, testData()];
On the above example, a single element of testArray can hold multiple types of data like integer, string, JSON object, float, and last we call the function inside an array.
Can we remove a specific elements from an array?
Answer is yes, We can remove element from array.
There is multiple way we can achieve this solution. Let start with first.
Solution #1
// ECMAScript 5 code
var removeValue = 6;
var fullArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
fullArray = fullArray.filter(function(item) {
return item !== removeValue
})
console.log(fullArray)
// [ 1, 2, 4, 5, 7, 8, 9, 10 ]
// ECMAScript 6 code
var fullArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
fullArray = fullArray.filter(item => item !== value)
console.log(fullArray)
// [ 1, 2, 4, 5, 7, 8, 9, 10 ]
Explain: On the above example we use the filter function of javascript. The filter function can return an array. Inside return function, we can put our logic with the element that is allowed and which is not allowed. Filter functions iterate every element so it’s easy to work and check every element from the array. We can also put a condition for multiple-element remove from array.
let multipleEle = [4, 5, 6]
var fullArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
fullArray = fullArray.filter(item => !multipleEle.includes(item))
console.log(fullArray)
// [ 1, 4 ]
The above logic you can use includes for checking item exists or not based on include response we decide element is in or not.
Solution #2
Old solution also apply for remove element using for loop.
var item = 4;
var newArr = [];
var arr = [1, 2, 3, 4, 5, 6];
for (var i = 0; i < arr.length; i++) {
if (item !== arr[i]) {
newArr.push(arr[i]);
}
}
console.log(newArr)
Maybe no need to give batter understanding of above example.
Let take one complex example to remove the JSON element from an array. Because users always try to call API in front-end, Sometimes we remove the row, element, or item from the array we have easy trick and tips for it.
let testArrayObject = [
{name: "Javascript everywhere", public: "20k+", daily_visitor: 9000},
{name: "Jsgrip", public: "22k+", daily_visitor: 5000},
{name: "Javascript Developer", public: "25k+", daily_visitor: 3000},
{name: "jsgrip.com", public: "100k+", daily_visitor: 10000},
{name: "Oyy teri", public: "2k+", daily_visitor: 10},
]
// Remove those record from array who has less then 5000 daily_visitor
Simple way one
testArrayObject = testArrayObject.filter(record => record.daily_visitor >= 5000);
console.log(testArrayObject)
Way two using underscore js or lodash js
testArrayObject = _.select(testArrayObject, function(data){
return testArrayObject.daily_visitor > 5000
});
console.log(testArrayObject)
If you any confusion related to this article, you can feel free to contact us