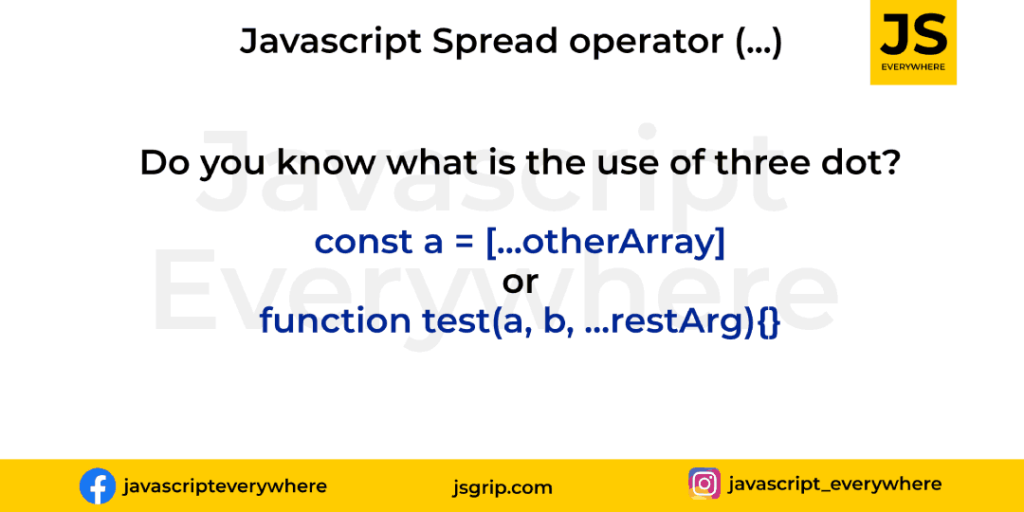
Unbelievable Feature release by ES6. it’s called spread operator or rest operator
The spread operator is used for spread syntax. This operator use in array and object. We also called spread elements
Let’s deep dive into all features. ES6 provides many operators and feature but some beginners are failed to remember this feature. From many experience developer also not using this feature during development
1) Copy array element using spread element
Do you want to copy array element into another variable, Or added extra element with copy let’s take an example
const testArray = [1, 2, 3, 4, 5, 6];
const arrayTwo = [...testArray];
console.log(testArray);
console.log(arrayTwo);
Above example is just copy one element to other example using spread element
const testJs = [
"Javascript", "Developer",
"jsgrip",
"js developer",
"hello all"
];
const extraElementJs = [...testJs, "Javascript everywhere"];
console.log(testJs);
console.log(extraElementJs);
In the above example, we copy elements with add one extra element. Add more element separate by commas
It’s interesting, do you like rest operator?
2) spread operator with function
Sometimes we have created multiple methods for different argument, for example, what happens when we create a method for the sum of value
function sum1(arg1, arg2) {
return arg1 + arg2;
}
function sum1(arg1, arg2, arg3) {
return arg1 + arg2 + arg3;
}
function sum1(arg1, arg2, arg3, arg4) {
return arg1 + arg2 + arg3 + arg4;
}
function sum1(arg1, arg2, arg3, arg4, arg5) {
return arg1 + arg2 + arg3, arg4 + arg5;
}
Just thinking what happen if argument is not specified.
Solution for above issue is using spread syntax
function sumOfArg(value, ...arg) {
// Write a sum logic of value here
}
3) You can use rest operator with Maths function as well
console.log(Math.max(...[1, 2, 3, 4, 5, 5, 6, 7, 8, 9, 10]));
console.log(Math.min(...[1, 2, 3, 4, 5, 5, 6, 7, 8, 9, 10]));
All above example and syntax you can use with object as well, like
const restTest = { name: "javascript everywhere", like: 110000 };
const restPage = { name: "javascript everywhere", follower: 200000 };
const obj = {...restTest}
console.log(obj);
const mergeObj = {...restPage, ...restTest};
console.log(mergeObj);
1) End of function argument you call rest parameter
2) A function or any other it’s called spread syntax
If you any comments or questions you can feel free to ask here. You can also ready array article as we create, remove a specific element from array