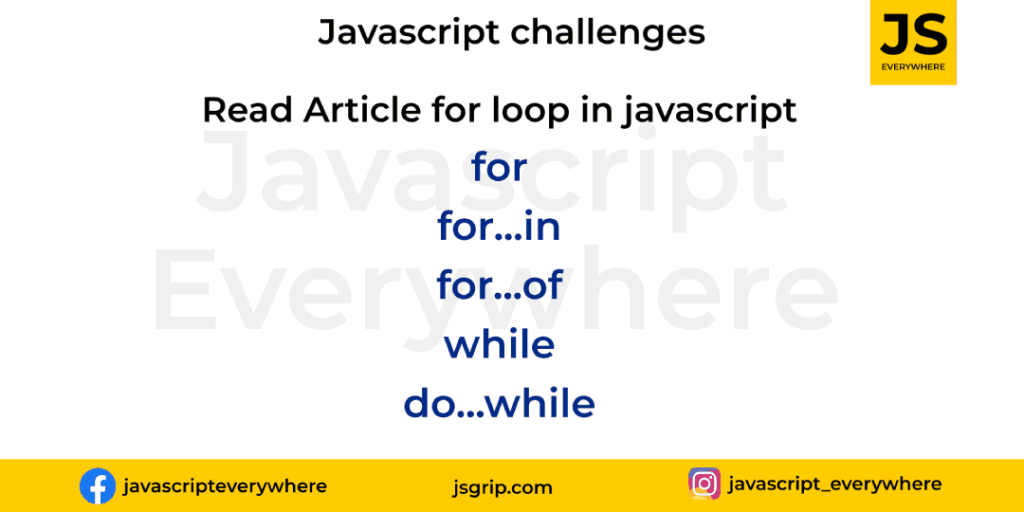
Loop executes the same code of block again and again. Loop is a control flow statement for specifying iteration, which allows code to be executed repeatedly. Repetitive task within a program to save time and effort.
Mainly javascript support 5 types of loops:
for
for…in
for…of
While
do…while
Don’t miss bonus tips at last it’s very useful for looping.
For Loop
For loop is control flow statement for specifying an iteration. Which allow to execute code in repeated manner.
//Syntax:
for(Declaration; condition; Increment / decrement){
// Your Logic here
// Statement
}
1) Declaration: It’s used for variable Declared or counter variable, Many developers said the scope of the loop as well.
2) Condition: Execution of loop is base on condition. If condition is true code is executed. Stop execution if the condition is false.
3) expression: Expression run after every iteration it might be Increment or decrement.
4) Statement: This is the body of for loop it executes if the condition is satisfied.
let arrayStr = "jsgrip.com".split();
for(let i = 0; i < arrayStr.length; i++){
console.log(arrayStr[i].toLocaleUpperCase())
}
// Output is JSGRIP.COM
You can write multiple Declaration, condition, or expression as well. Check the below example.
for(let i = 0, j = 6; i <= 6, j >= 0; i++, j--){
if (i == j){
console.log("Javascript everywhere");
}
}
// Output is javascript everywhere only one time
For…in
For..in statement basally uses for enumerable properties of an object. Each distinct key assigns to variable and base on key we can access the value of that key from an object. let check syntax and example for batter understanding.
// Syntax
for (variable in object) {
// Statement
}
//Example:
const dataArray = {
name: 'Javascript everywhere',
like: 5689565,
follower: 64895656,
platform: 'facebook/instagram'
}
for (const key in dataArray){
console.log(key);
}
// Output is
// name
// like
// follower
// platform
const dataArray = {
name: 'Javascript everywhere',
like: 5689565,
follower: 64895656,
platform: 'facebook/instagram'
}
for (const key in dataArray){
console.log(key + " : " + dataArray[key]);
}
// Output is
// name : Javascript everywhere
// like : 5689565
// follower : 64895656
// platform : facebook/instagram
developer ask me a question regarding the for-in loop, the question is can we use for-in with an array? Yes, We can use it. Index of array considers as keys. Let’s take an example.
let arrayVals = ['javascript everywhere', 'jsgrip.com'];
arrayVals['popularLanguage'] = 'Javascript';
for (const key in arrayVals){
console.log(key + " : " + arrayVals[key]);
}
// Output is
// 0 : javascript everywhere
// 1 : jsgrip.com
// popularLanguage : Javascript
For…of
In ES6 introduce a new loop called for…of. This loop is used for iterate over an array or iterable objects like a string.
//Syntax:
for(variable of arrayObject){
// statement
}
// Example
let arrayVals = [
'javascript everywhere',
'jsgrip.com',
'Javascript for developer',
'Help to developer',
'professional developers are alwasy help each other'
];
for (const singleVal of arrayVals){
console.log(singleVal);
}
// Output is
// Each array element print one by one
Very important: You can not use for…of for the object. The object is not iterable. You can go with for-in.
While
Evaluating the condition at the starting of the loop. the loop executes base on condition is true or false. You can say the entry control loop as well. The statement is not executed if the condition returns false.
// syntax
while (condition){
// statement
}
// Example
let val = 0;
while (val != 5) {
console.log(val); // Output is 1 2 3 4 5
val++;
}
do…while loop
While loop and do loop are the same. Just different if check condition after executing the statement. The developer said it’s an exit control loop.
// syntax
do {
// statement
} while (condition)
// Example
let val = 0;
do {
val++;
console.log(val); // Output is 1 2 3 4 5
} while (val != 5);
Bonus Tips: With loop you can use two statement break and continue.
Break: The statement is used to break the loop or stop execution in between.
Continue: Continue statement use for skip one single iteration from the loop and execute the next one.
for(let a = 0; a < 10; a++){
if (a == 5){
continue;
}
if (a == 7){
break;
}
console.log(a);
}
// Output is
// 0
// 1
// 2
// 3
// 4
// 6
If you have any comment and suggestion you can ask us anytime. Do you know what is the use of arrow function?